Iterator和Enumeration的重要区别:
1:Enumeration中没有删除方法,只有遍历。
2:Enumeration是先进后出,而Iterator是先进先出。
虽然两个接口的功能是重复的,
但是Iterator由于比Enumeration多了remove方法且接口方法名字更短,
因此推荐在后面的类中使用Iterator
迭代器将遍历序列的操作和序列底层(存储结构)相分离。可以说:迭代器统一了对容器的访问方式,而不用在乎容器的结果
使用方法iterator()要求容器返回一个Iterator。Iterator将准备好返回序列的第一个元素; 使用next()获取序列中下一个元素; 使用hasNext()检查序列中是否有元素; 使用remove()将迭代器新返回的元素删除。
Iterator与Enumeration的区别
Enumeration的速度是Iterator的两倍,也使用更少的内存。Enumeration是非常基础的,也满足了基础的需要。 但是,与Enumeration相比,Iterator更加安全,因为当一个集合正在被遍历的时候,它会阻止其它线程去修改集合。
迭代器取代了Java集合框架中的Enumeration。迭代器允许调用者从集合中移除元素,而Enumeration不能做到。为了使它的功能更加清晰,迭代器方法名已经经过改善。
Iterator 和 Eumberation都是Collection集合的遍历接口,我们先看下他们的源码接口
package java.util; public interface Enumeration<E> { boolean hasMoreElements(); E nextElement(); }
package java.util; public interface Iterator<E> { boolean hasNext(); E next(); void remove(); }
1 引入的时间不同
Iteration是从JDK1.0 开始引入,Enumeration是从JDK1.2开始引入
2 接口不同
Enumeration只有2个函数接口。通过Enumeration,我们只能读取集合的数据,而不能对数据进行修改
Iteration只有3个函数的接口。Iteration除了能读取集合的数据之外,也能对数据进行删除
Java的API 解析里面对两个接口的不同是这样解释的:
Iterators differ from enumerations in two ways:
- Iterators allow the caller to remove elements from the underlying collection during the iteration with well-defined semantics.
- Method names have been improved.
The bottom line is, both Enumeration
and Iterator
will give successive elements, but Iterator
is improved in such a way so the method names are shorter, and has an additional remove
method. Here is a side-by-side comparison:
Enumeration Iterator ---------------- ---------------- hasMoreElement() hasNext() nextElement() next() N/A remove()
3 Enumeration是历史遗留接口
Enumeration接口作为一个遗留接口主要用来实现例如 Vextor HashTable Stack 的遍历,而Iterator作为比较新的接口,实现了Collection框架下大部分实现类的遍历比如说 ArrayList LinkedList HashSet LinkedHashSet TreeSet HashMap LinkedHashMap TreeMap 等等
4 Fail-Fast Vs Fail-Safe
Iterator支持Fail-Fast机制,当一个线程在遍历时,不允许另外一个线程对数据进行修改(除非调用实现了Iterator的Remove方法)。、
因此Iterator被认为是安全可靠的遍历方式
5、应该使用哪个
还是摘一段JAVA API解析上的一段话(大意就是虽然两个接口的功能是重复的,但是Iterator由于比Enumeration多了remove方法且接口方法名字更短,因此推荐在后面的类中使用Iterator)
According to Java API Docs, Iterator is always preferred over the Enumeration. Here is the note from the Enumeration Docs.
NOTE: The functionality of this interface is duplicated by the Iterator interface. In addition, Iterator adds an optional remove operation,
and has shorter method names. New implementations should consider using Iterator in preference to Enumeration.
Iterator接口的功能是遍历Collection容器中的元素。
方法:
boolean hasNext():用来判断当前游标后面是否还存在元素,如果存在就返回true,不存在就返回false。
Object next():先返回当前游标右边的元素,然后游标后移一个位置。
void remove():删除最近返回的元素。
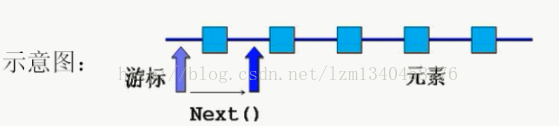
从示意图中我们可以看出:游标最开始的位置是在第一个元素的前面。
下面通过一个实例来说明:
public class IteratorTest { public static void main(String[] args) { /*创建一个list*/ List list = new ArrayList(); list.add(1); list.add(2); list.add(3); list.add("廖泽民"); list.add("abc"); /*调用迭代器方法遍历集合元素*/ displayByIterator(list); } /** * 通过Iterator遍历所有的Collection接口的实现类 */ public static void displayByIterator(Collection collection){ /*创建一个迭代器*/ Iterator it = collection.iterator(); /*遍历*/ while (it.hasNext()){ /*打印元素*/ System.out.println(it.next()); } } }
Enumeration和Iterator一样都是遍历集合。
Enumeration中的两个方法:
boolean hashMoreElements():判断是否有更多的元素可以提取,如果有的话就返回true,否则返回false。
Object nextElement():如果至少存在一个可提供的元素,则返回此枚举的下一个元素。
示例:
public class EnumerationTest { public static void main(String[] args) { /*创建一个hashTable*/ Hashtable hashtable = new Hashtable(); /*向hashTable中添加数据*/ hashtable.put("id", 1); hashtable.put("name", "廖钟民"); hashtable.put("sex", "男"); /*调用elements()方法将table转换为Enumeration集合*/ Enumeration enumeration = hashtable.elements(); /*.hashElemnents()判断是否存在更多的元素*/ while (enumeration.hasMoreElements()){ /*输出*/ System.out.println(enumeration.nextElement()); } } }