一、什么是结构化命令?
许多程序要求对shell脚本中的命令施加一些逻辑流程控制。有一类命令会根据条件使脚本跳过某些命令。这样的命令通常称为结构化命令。
二、使用逻辑化命令的好处
恰当的使用结构化命令,会使我们的代码看起来更有逻辑性、可读性。会让我们的工作变得更加轻松。
三、逻辑化命令if、for、while、until的格式
阅读目录
if-then语句
bash shell的if语句会执行if后面的那个命令,如果该命令的退出码状态为0会执行then部分的命令,如果是其他值不会执行。
格式如下:
if command then commands fi
实例:
[root@node1 ljy]# more ceshi.sh #!/bin/bash if pwd then echo "ok" fi [root@node1 ljy]# sh ceshi.sh /ljy ok
在then部分可以使用多条命令。
[root@node1 ljy]# more ceshi.sh #!/bin/bash testuser=ljy if grep $testuser /etc/passwd then echo "ok" fi [root@node1 ljy]# sh ceshi.sh ljy:x:1000:1000::/home/ljy:/bin/bash ok
if-then-else
格式如下:
if command then commands else commands fi
用法很简单,看一个例子就行
[root@node1 ljy]# more ceshi.sh #!/bin/bash testuser=ljy if grep $testuser /etc/passwd then echo "$testuser exit on system!" else echo "$testuser does ont on system!" fi [root@node1 ljy]# sh ceshi.sh ljy:x:1000:1000::/home/ljy:/bin/bash ljy exit on system! #此时我定义一个不存在的变量 [root@node1 ljy]# more ceshi.sh #!/bin/bash testuser=ljy1 if grep $testuser /etc/passwd then echo "$testuser exit on system!" else echo "$testuser does ont on system!" fi [root@node1 ljy]# sh ceshi.sh ljy1 does ont on system!
嵌套if
语法很简单看一个例子:
[root@node1 ljy]# more ceshi.sh #!/bin/bash testuser=zhangsan if grep $testuser /etc/passwd then echo "$testuser exit on system!" else echo "$testuser does ont on system!" if ls -d /home/$testuser then echo "but $testuser have a directory!" fi fi [root@node1 ljy]# sh ceshi.sh zhangsan does ont on system! /home/zhangsan but zhangsan have a directory!
也可以用else部分的另外一种形式elif
格式如下:
if command then commands elif command2 then more commands fi
实例:
[root@node1 ljy]# more ceshi.sh #!/bin/bash testuser=zhangsan if grep $testuser /etc/passwd then echo "$testuser exit on system!" elif ls -d /home/$testuser then echo "but $testuser have a directory!" fi [root@node1 ljy]# sh ceshi.sh /home/zhangsan but zhangsan have a directory!
test命令
如果test命令中列出的条件成立,test命令就会退出并返回特推出状态码0
test 命令可以判断3类条件:
1. 数值比较
2. 字符串比较
3. 文件比较
1、数值比较
注意:test 命令中不能使用浮点数。
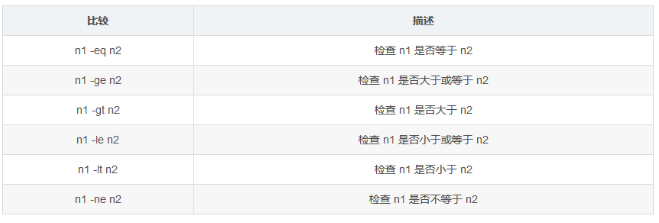
实例:
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1=10 value2=11 # if [ $value1 -gt 5 ] #左括号右侧和右括号左侧各加一个空格,否则会报错。 then echo "$value1 is bigger than 5" fi [root@node1 ljy]# sh ceshi.sh 10 is bigger than 5
2、字符串比较
条件测试还允许比较字符串值
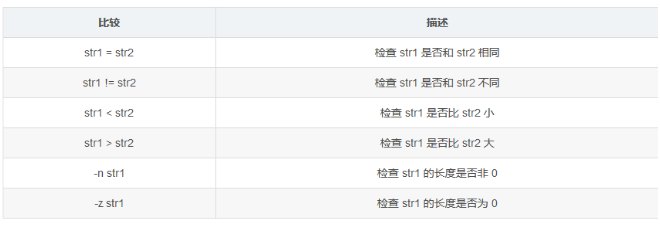
字符串比较的三大注意事项:
1. 比较的变量最好加上双引号。
2. 大于小于符号必须转义(使用\>),否则 shell 会把它们当做重定向符号而把字符串值当做文件名。
3. 大于小于顺序和 sort 命令所采用的不同。(test默认大写字母小于小写字母)
实例:
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1=basketball value2=football # if [ $value1 \> $value2 ] then echo "$value1 is greater than $value2" else echo "$value1 is less than $value2" fi [root@node1 ljy]# sh ceshi.sh basketball is less than football
-n和-z可以检查一个变量是否含有数据。
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1=basketball value2=' ' # if [ -n $value1 ] then echo "'$value1' is not empty" else echo "'$value1' is empty" fi # if [ -z $value2] then echo "'$value2' is empty" else echo "'$value2' is not empty" fi [root@node1 ljy]# sh ceshi.sh 'basketball' is not empty ' ' is empty
-n判断长度是否非0,-z判断长度是否为0
3、文件比较
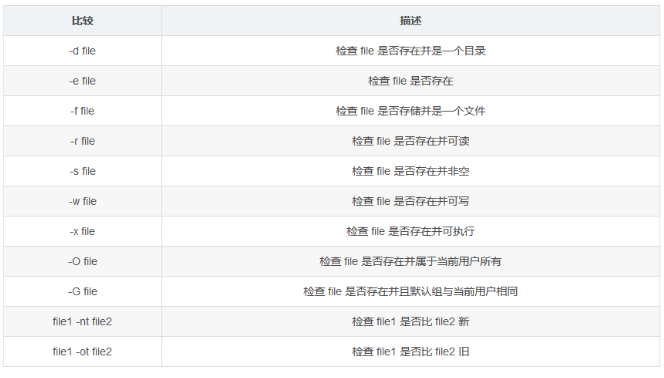
-d检测目录是否存在。
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1=/home/ljy if [ -d $value1 ] then echo "$value1 is exited" else echo "$value1 is not exited" fi [root@node1 ljy]# sh ceshi.sh /home/ljy is exited
-e允许脚本代码在使用文件或者目录前先检测是否存在
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1='lisi' if [ -e /home/$value1 ] then echo "$value1 is exited" else echo "$value1 is not exited" fi [root@node1 ljy]# sh ceshi.sh lisi is exited
-f确定对象是否为文件
[root@node1 ljy]# more ceshi.sh #!/bin/bash value1='zhangsan' if [ -e /home/$value1 ] #判断变量是否存在 then echo "$value1 is exited" if [ -f /home/$value1 ] #判断是否为文件 then echo "$value1 is a file" else echo "$value1 is a directory" fi else echo "$value1 is not exited" fi [root@node1 ljy]# sh ceshi.sh zhangsan is exited zhangsan is a directory
-r测试文件是否可读
[ljy@node1 ljy]$ more ceshi2.sh #!/bin/bash pwfile=/home/lisi # if [ -r $pwfile ] then tail $pwfile else echo "this file unable to read!" fi [ljy@node1 ljy]$ sh ceshi2.sh this file unable to read!
-s检测文件是否为非空,尤其是在不想删除非空文件的时候。
[root@node1 ljy]# more ceshi2.sh #!/bin/bash pwfile=/home/lisi # if [ -s $pwfile ] then echo "this file is not empty" else echo "$pwfile is empty" echo "Deleting empty file..." rm $pwfile fi [root@node1 ljy]# sh ceshi2.sh /home/lisi is empty Deleting empty file...
-w判断对文件是否可写。
[root@node1 ljy]# more ceshi2.sh #!/bin/bash pwfile=/home/lisi # if [ -w $pwfile ] then echo "this file can be write!" date +%H%M >> $pwfile else echo "$pwfile can not be write" fi [root@node1 ljy]# sh ceshi2.sh this file can be write!
-x判断文件是否有执行权限。
当然这是针对的非root用户。
[root@node1 ljy]# more ceshi2.sh #!/bin/bash pwfile=/home/test.sh # if [ -x $pwfile ] then echo "this file can be run!" sh $pwfile else echo "$pwfile can not be run!" fi [root@node1 ljy]# sh ceshi2.sh this file can be run!
复合条件测试
if-then 语句允许使用布尔逻辑来组合测试:
– 与:[ condition1 ] && [ condition2 ] 或者 [ condition1 -a condition2 ]
– 或:[ condition1 ] || [ condition2 ] 或者 [ condition1 -o condition2 ]
– 非:[ !condition ]
实例:
[root@node1 ljy]# more ceshi2.sh #!/bin/bash pwfile=/home/test.sh # if [ -d $pwdfile ] && [ -x $pwfile ] then echo "this file can be run!" sh $pwfile else echo "$pwfile can not be run!" fi [root@node1 ljy]# sh ceshi2.sh this file can be run!
case命令
为单个变量寻找特定的值,可以用 case 命令,而不是写那么多的 elif 语句检查。case 命令会检查单个变量列表格式的多个值:
case variable in pattern1 | pattern2) commands1 ;; pattern3) commands2 ;; *) default commands ;; esac
case 命令会将指定的变量同不同模式进行比较。
如果变量和模式是匹配的,那么 shell 会执行为该模式指定的命令。
也可以通过竖线操作符来分割模式,在一行列出多个模式。星号会捕获所有跟所有列出的模式都不匹配的值。
[root@node1 ljy]# more ceshi2.sh #!/bin/bash case $USER in root | barbara) echo "Welcome $USER" echo 'Enjoy your visit' ;; testing) echo "Special testing acount" ;; jessica) echo "Don't forget to log off" ;; *) echo "Sorry, you aren't allowed here" ;; esac [root@node1 ljy]# sh ceshi2.sh Welcome root Enjoy your visit